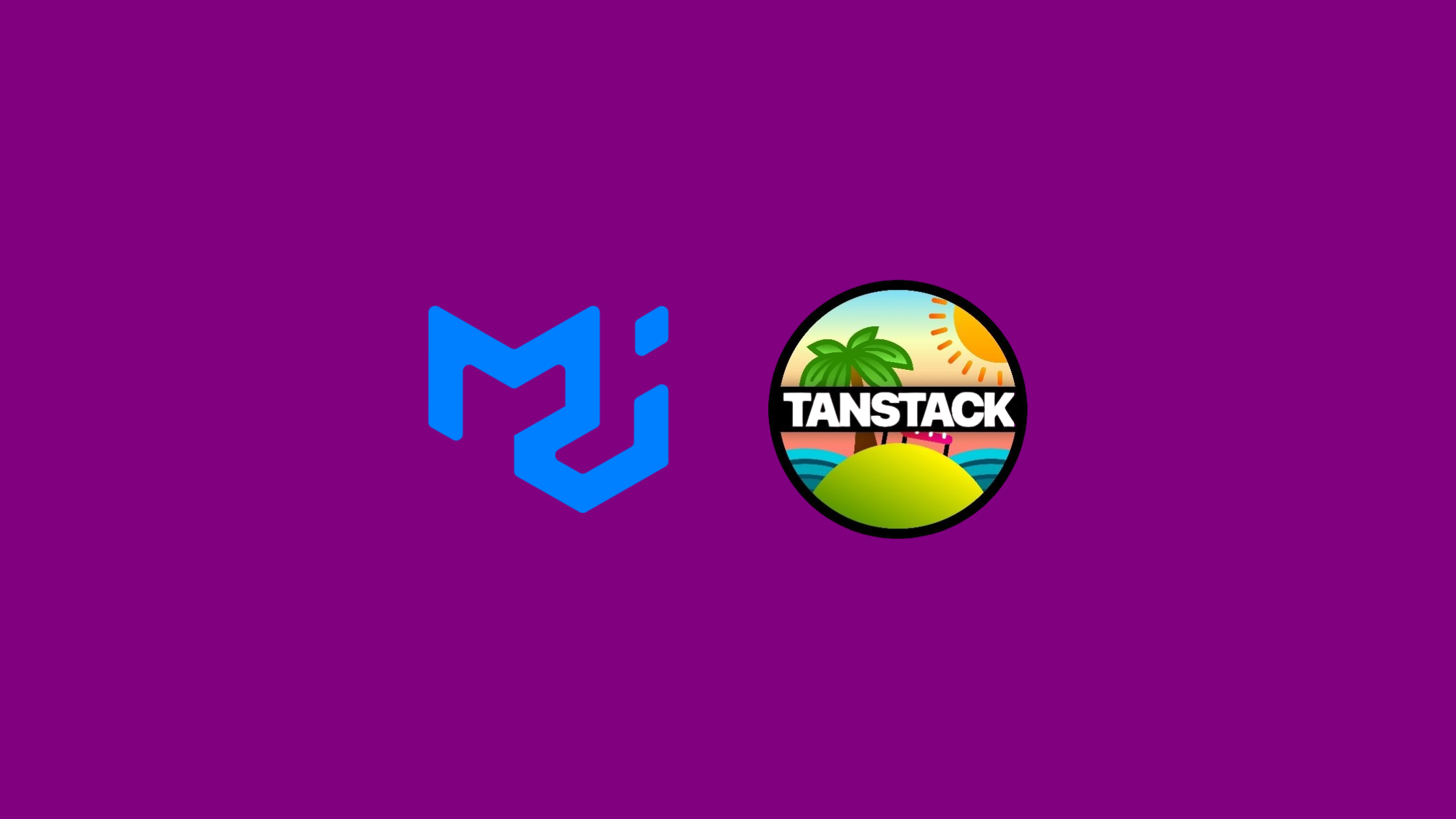

Data grids are everyday matter when building web applications. Two popular libraries for implementing data grids in React applications have emerged: MUI Data Grid and TanStack Table. This article will explore how to implement these two options, comparing their features and ease of use.
MUI Data Grid
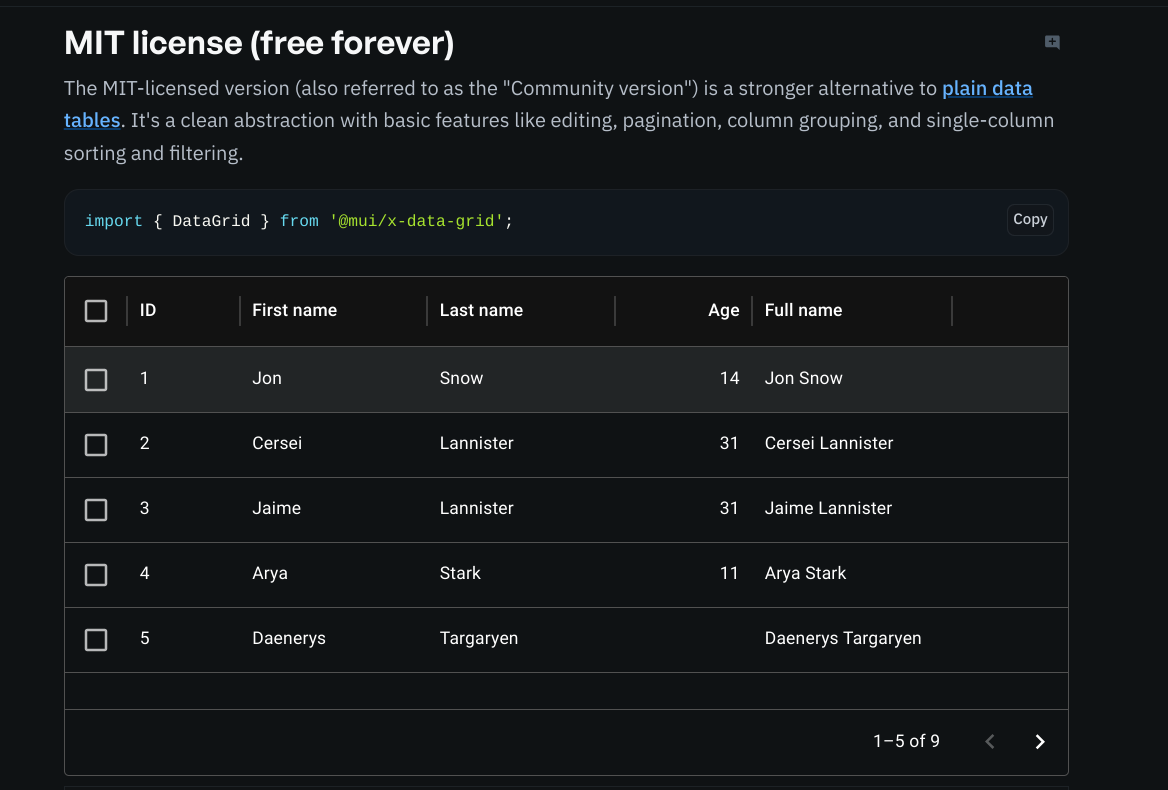
MUI Data Grid is a rich component built on top of Material-UI, designed for high performance and flexibility. It comes in three versions: Community (MIT licensed), Pro, and Premium. The Community version supports basic functionalities like sorting, pagination, and editing, while the Pro and Premium versions offer advanced features such as row grouping, aggregation, and Excel export capabilities.
Getting Started with MUI Data Grid
To implement MUI Data Grid, follow these steps.
1. Installation: Install the package using npm:
npm install @mui/x-data-grid
2. Importing the Component: Import the DataGrid
from the library:
import { DataGrid } from '@mui/x-data-grid';
3. Defining Rows and Columns: Create an array of row objects and define the columns:
const rows = [
{ id: 1, col1: 'Hello', col2: 'World' },
{ id: 2, col1: 'DataGrid', col2: 'is Awesome' },
];
const columns = [
{ field: 'col1', headerName: 'Column 1', width: 150 },
{ field: 'col2', headerName: 'Column 2', width: 150 },
];
4. Rendering the Data Grid:
export default function App() {
return (
<div style={{ height: 300, width: '100%' }}>
<DataGrid rows={rows} columns={columns} />
</div>
);
}
TanStack Table
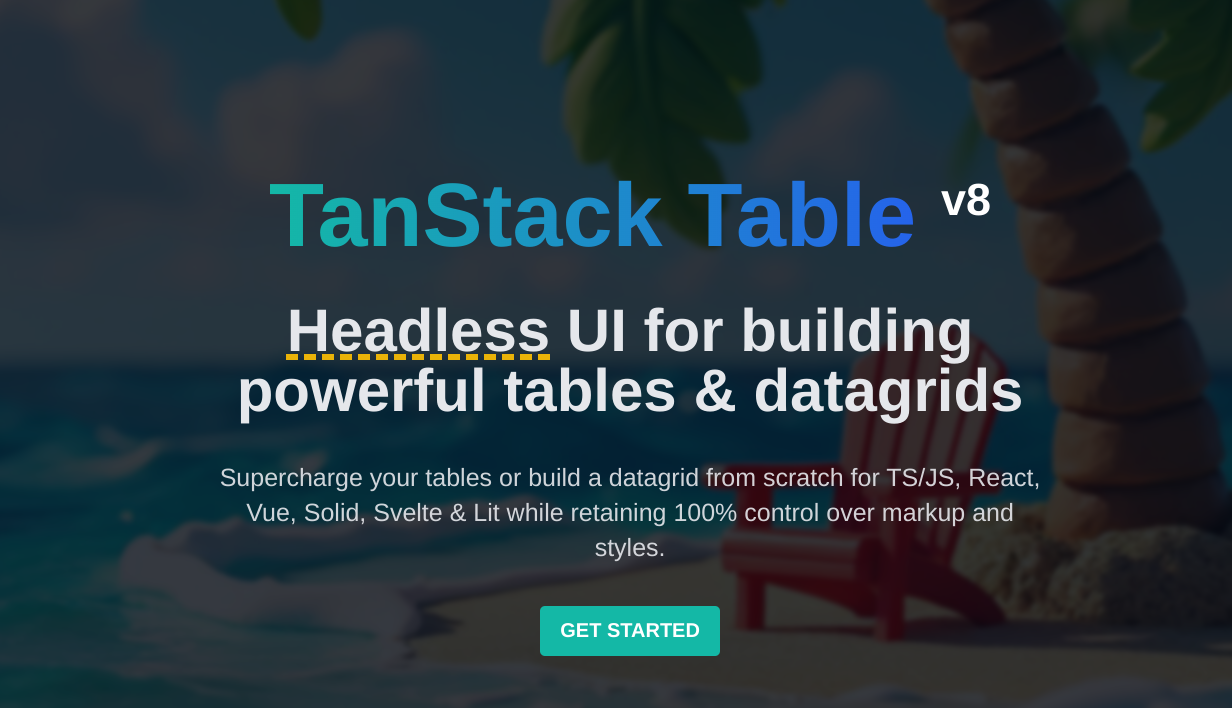
TanStack Table (used to be React Table) is a headless UI library that provides hooks to build tables without a predefined UI. This gives you full control on the appearance and behavior of their tables.
Getting Started with TanStack Table
To implement TanStack Table, follow these steps:
1. Installation:
npm install @tanstack/react-table
2. Creating a Table Instance:
import { useTable } from '@tanstack/react-table';
const data = [
{ col1: 'Hello', col2: 'World' },
{ col1: 'TanStack', col2: 'is Great' },
];
const columns = [
{ accessorKey: 'col1', header: 'Column 1' },
{ accessorKey: 'col2', header: 'Column 2' },
];
const table = useTable({ data, columns });
3. Rendering the Table:
export default function App() {
return (
<table>
<thead>
{table.getHeaderGroups().map(headerGroup => (
<tr key={headerGroup.id}>
{headerGroup.headers.map(column => (
<th key={column.id}>{column.renderHeader()}</th>
))}
</tr>
))}
</thead>
<tbody>
{table.getRowModel().rows.map(row => (
<tr key={row.id}>
{row.getVisibleCells().map(cell => (
<td key={cell.id}>{cell.renderCell()}</td>
))}
</tr>
))}
</tbody>
</table>
);
}
Comparison of Features
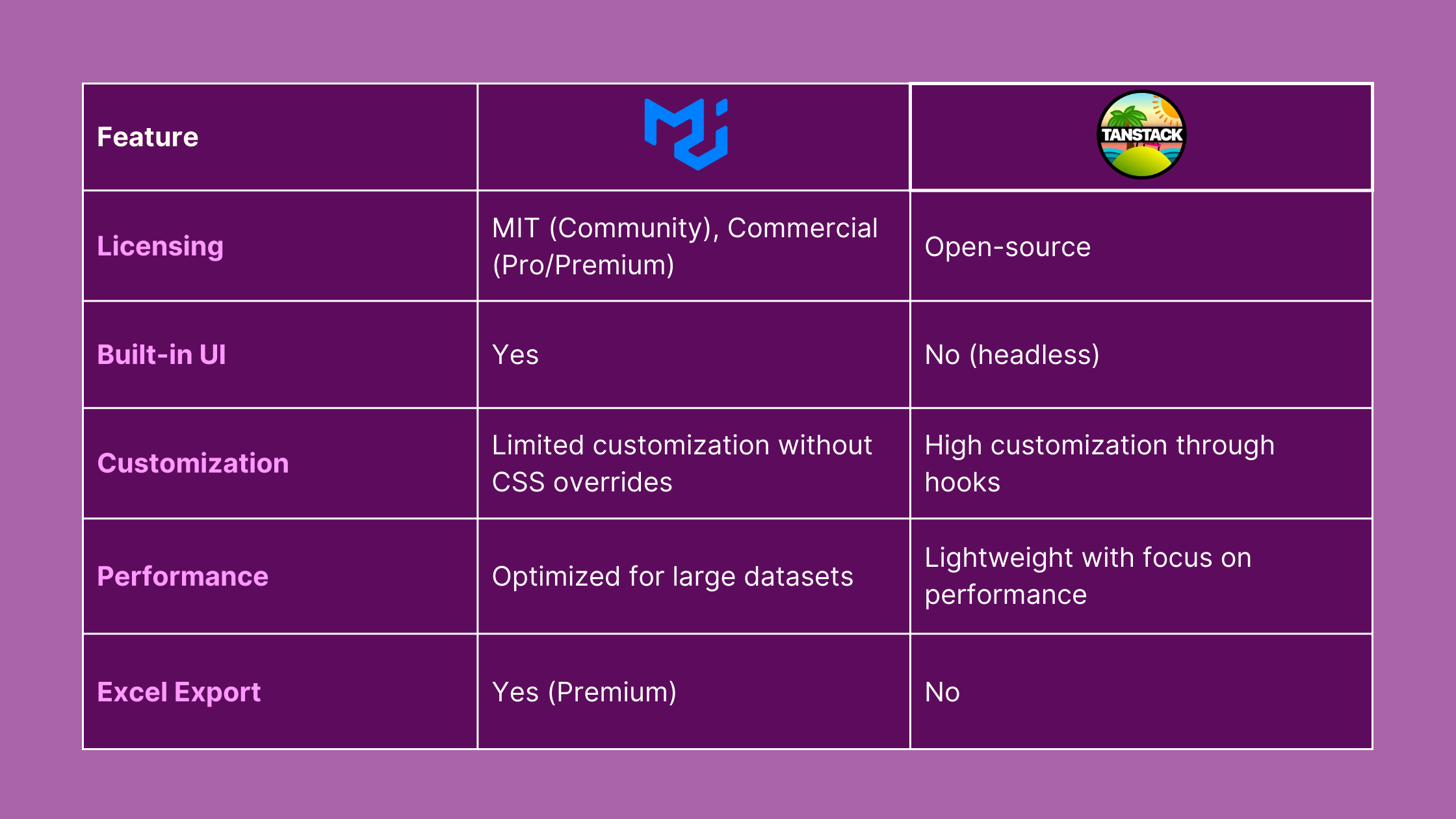
Which one to choose
Choosing between MUI Data Grid and TanStack Table depends on your project requirements:
- If you need an extensive product with built-in features and are willing to pay for advanced functionalities, MUI Data Grid is your go-to choice.
- If you prefer complete control without being tied to a specific UI framework, TanStack Table offers that kind of flexibility.
Both libraries have their strengths and can be effective tools for implementing data grids in React applications.
Have no time?
Creating UIs can be time consuming.
Particularly if you are implementing a Figma design.
Polipo is the first Figma compiler.
Curious to check it out.
Start playing.