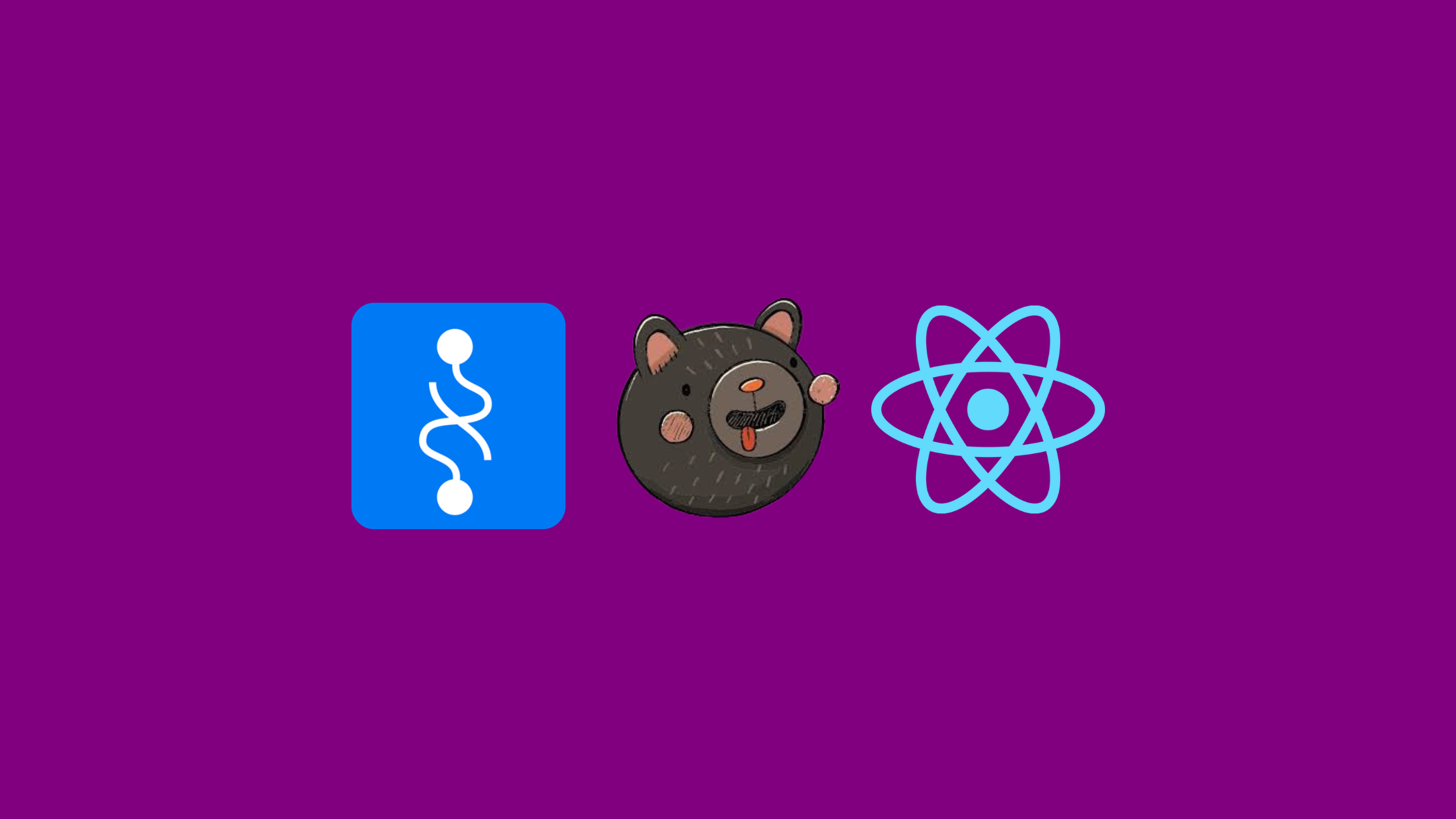

State management is a core part of building web applications, and in the React ecosystem (including Next.js), developers have several tools for state management. Among these, Recoil, Zustand, and the built-in Context API are popular choices, each with unique pros and cons.
In this article, we'll compare these tools based on their functionality, performance, and use cases, helping you decide the best option for your Next.js project.
General Overview
Recoil
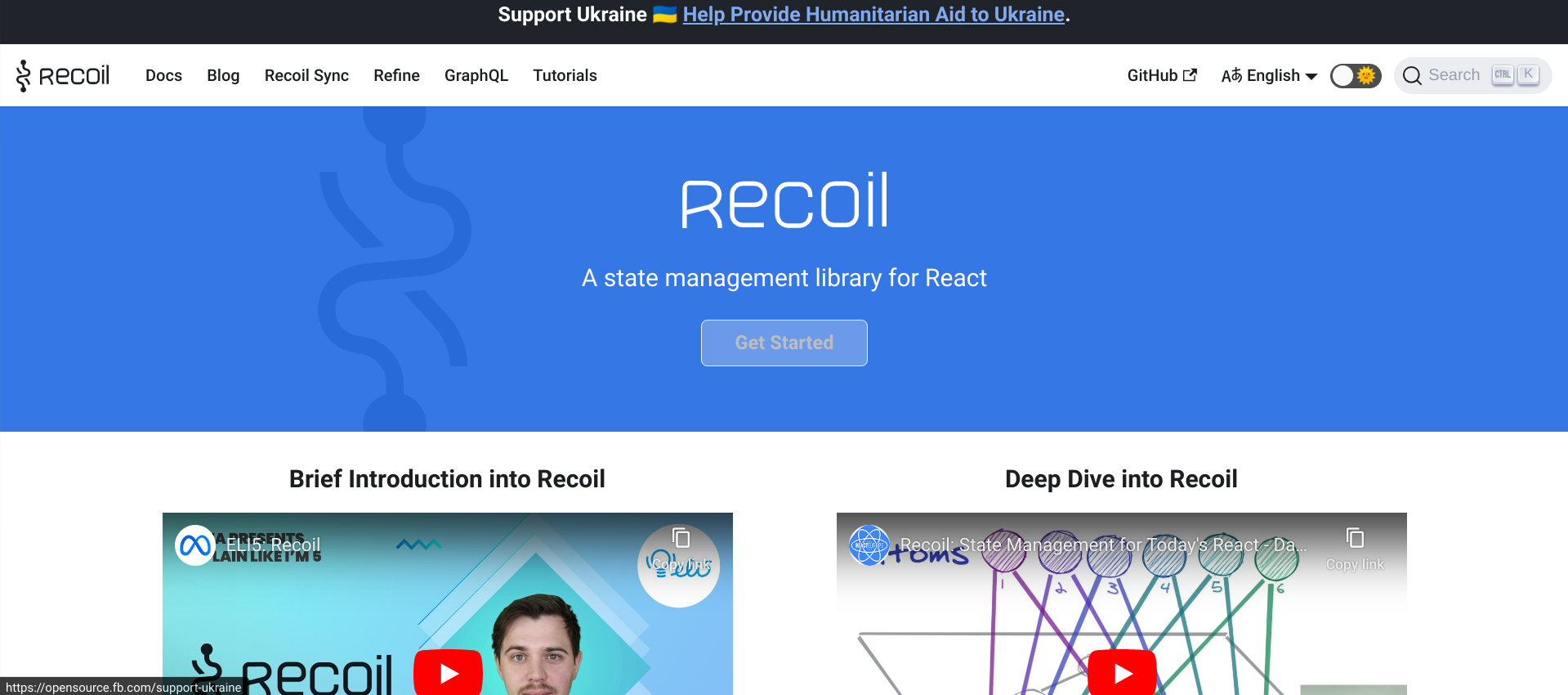
Recoil is a state management library created by Facebook. It provides a declarative, fine-grained approach to managing global state with features like derived state and subscriptions. Recoil integrates seamlessly with React, making it intuitive for developers already familiar with React hooks.
Key Features:
- Atom and selector-based state management.
- Reactivity with dependency tracking.
- Server-side rendering (SSR) support in Next.js.
- Easy to scale for complex applications.
Zustand
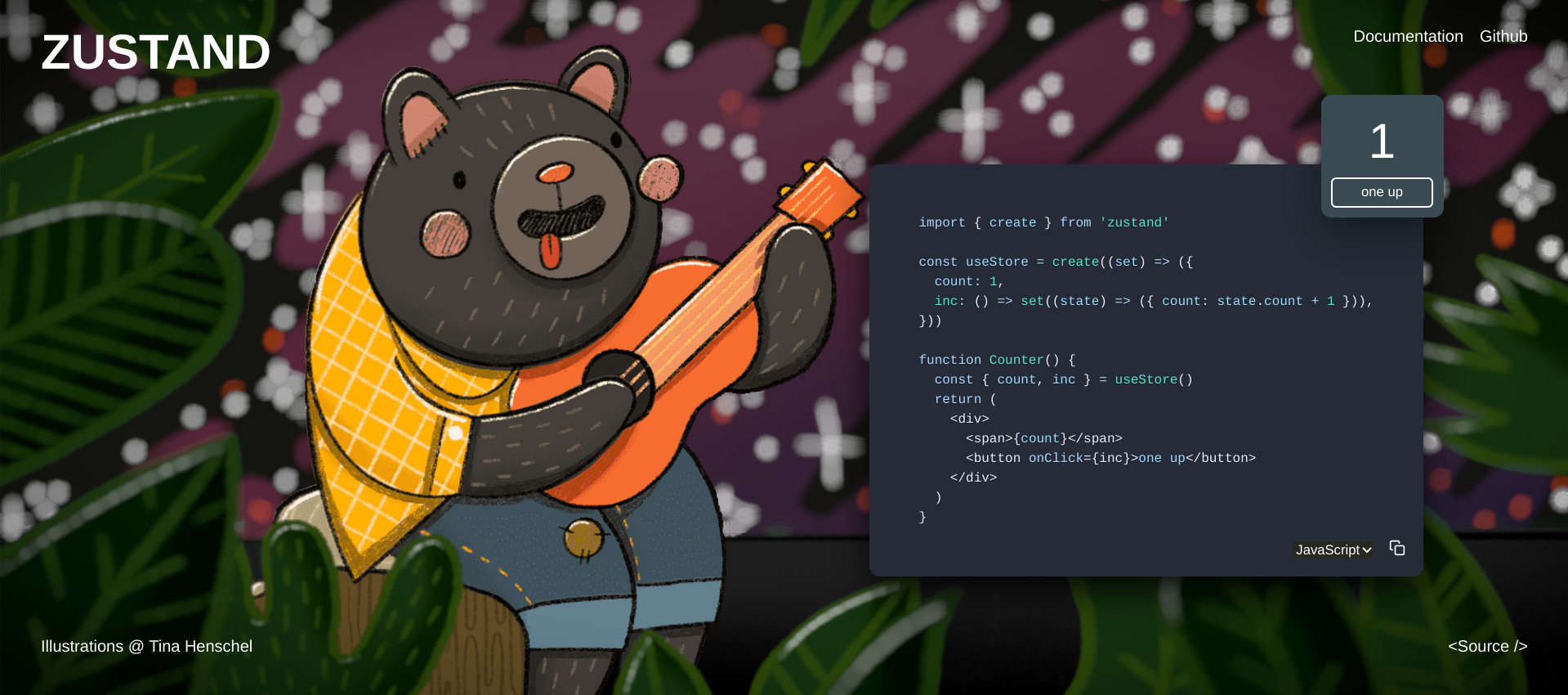
Zustand (German for "state") is a small, fast, and unopinionated state management library. It uses a simple API with a focus on minimizing boilerplate and maximizing performance. It is lightweight yet powerful.
Key Features:
- Minimal API with no boilerplate.
- Supports both global and local state.
- Highly performant with selective re-renders.
- Works great with Next.js.
Context API
The Context API is built into React, allowing you to share state between components without manually passing props through the component tree. While it isn't a full-fledged state management library, it can handle simple use cases effectively.
Key Features:
- Native to React, no additional dependencies.
- Simple and straightforward for small-scale state management.
- Works out-of-the-box with Next.js.
Comparison Based on Key Criteria
Performance
- Recoil: Uses granular subscriptions, ensuring components re-render only when their specific state changes. However, the performance might be affected in highly dynamic applications with complex dependency graphs.
- Zustand: Extremely fast due to its simplicity and optimized architecture. It minimizes re-renders by using selector-based state updates.
- Context API: Performance can degrade in larger applications due to re-rendering of all components that consume a given context. This can be mitigated using memoization techniques, but it adds some complexity.
Ease of Use
- Recoil: The atom-selector paradigm can have a learning curve but feels natural for React developers after initial setup.
- Zustand: Easy to set up and use, with a minimal API that doesn’t require extensive configuration.
- Context API: Simple to understand and use but can become cumbersome for complex state management involving multiple contexts.
Scalability
- Recoil: Designed for scalability with features like derived state and dependency tracking, making it ideal for large applications.
- Zustand: Scales well due to its lightweight nature and modular approach, but it may lack advanced features like dependency tracking out of the box.
- Context API: Limited scalability. Managing deeply nested or highly interdependent state using Context API can lead to "prop-drilling-like" issues with contexts.
SSR in Next.js
- Recoil: Offers built-in support for SSR, making it a solid choice for Next.js applications that rely on server-side rendering.
- Zustand: SSR support is possible but requires additional configuration to persist and hydrate state between server and client.
- Context API: Works natively with SSR in Next.js since it’s built into React, but additional setup may be required for state hydration.
Ecosystem and Community
- Recoil: Backed by Facebook, it has a growing community and robust documentation. However, it's still relatively new compared to older tools.
- Zustand: Community-driven with active development and support. It has a smaller but highly dedicated following.
- Context API: As part of React, it benefits from extensive documentation and support across the React community.
Use Cases for Next.js Projects
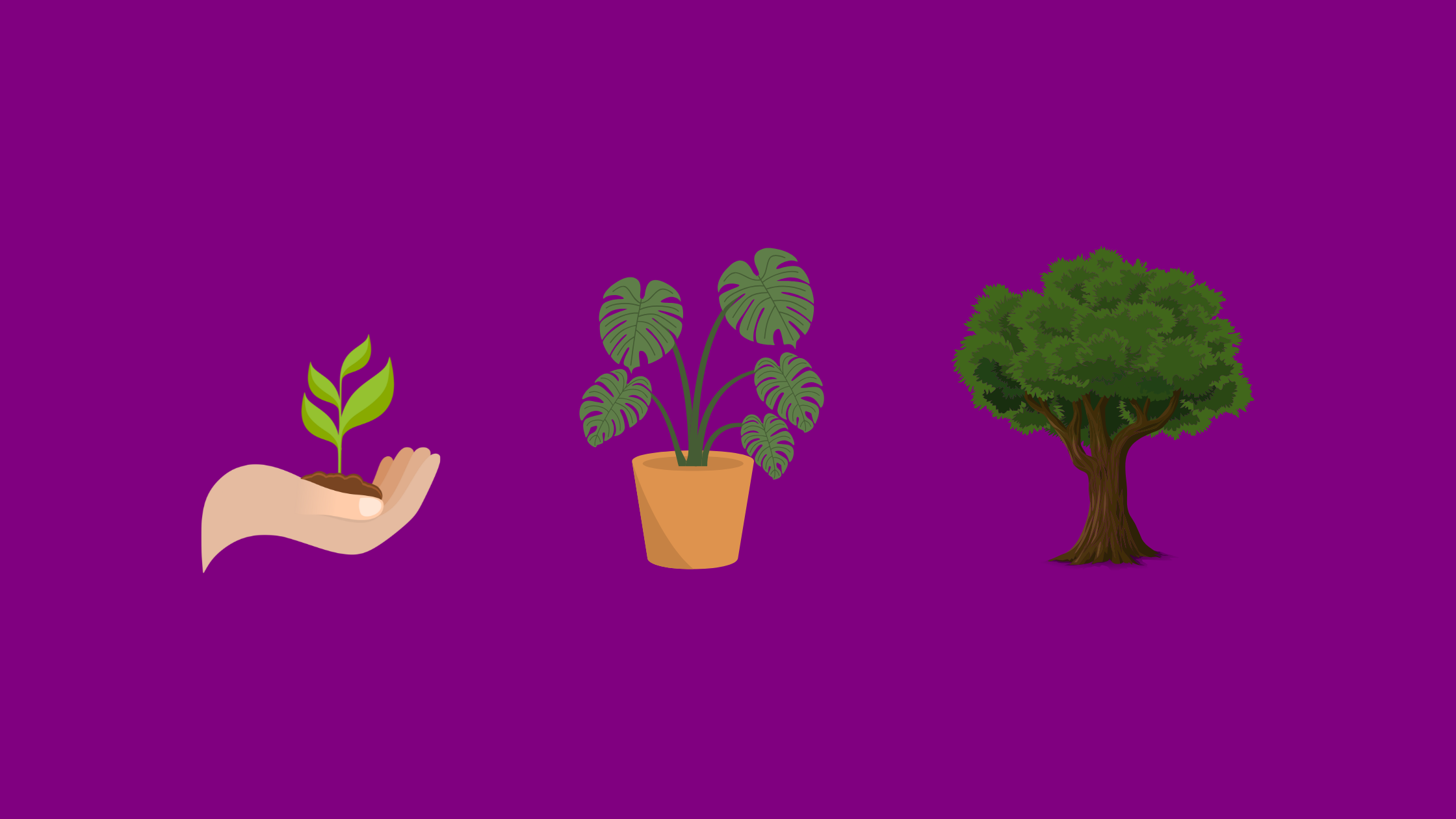
When to Use Recoil
- You’re building a medium-to-large application with complex state dependencies.
- Your app requires fine-grained reactivity (e.g., dashboards or collaborative tools).
- You want built-in SSR support for Next.js.
When to Use Zustand
- You need a lightweight, performant state manager.
- Your project demands minimal boilerplate with maximum flexibility.
- You want a small library footprint for optimal performance.
When to Use Context API
- Your application is small or medium-sized with limited global state requirements.
- You prefer built-in tools and want to avoid adding dependencies.
- Your team is familiar with React and prefers simplicity over advanced features.
Pros and Cons Summary
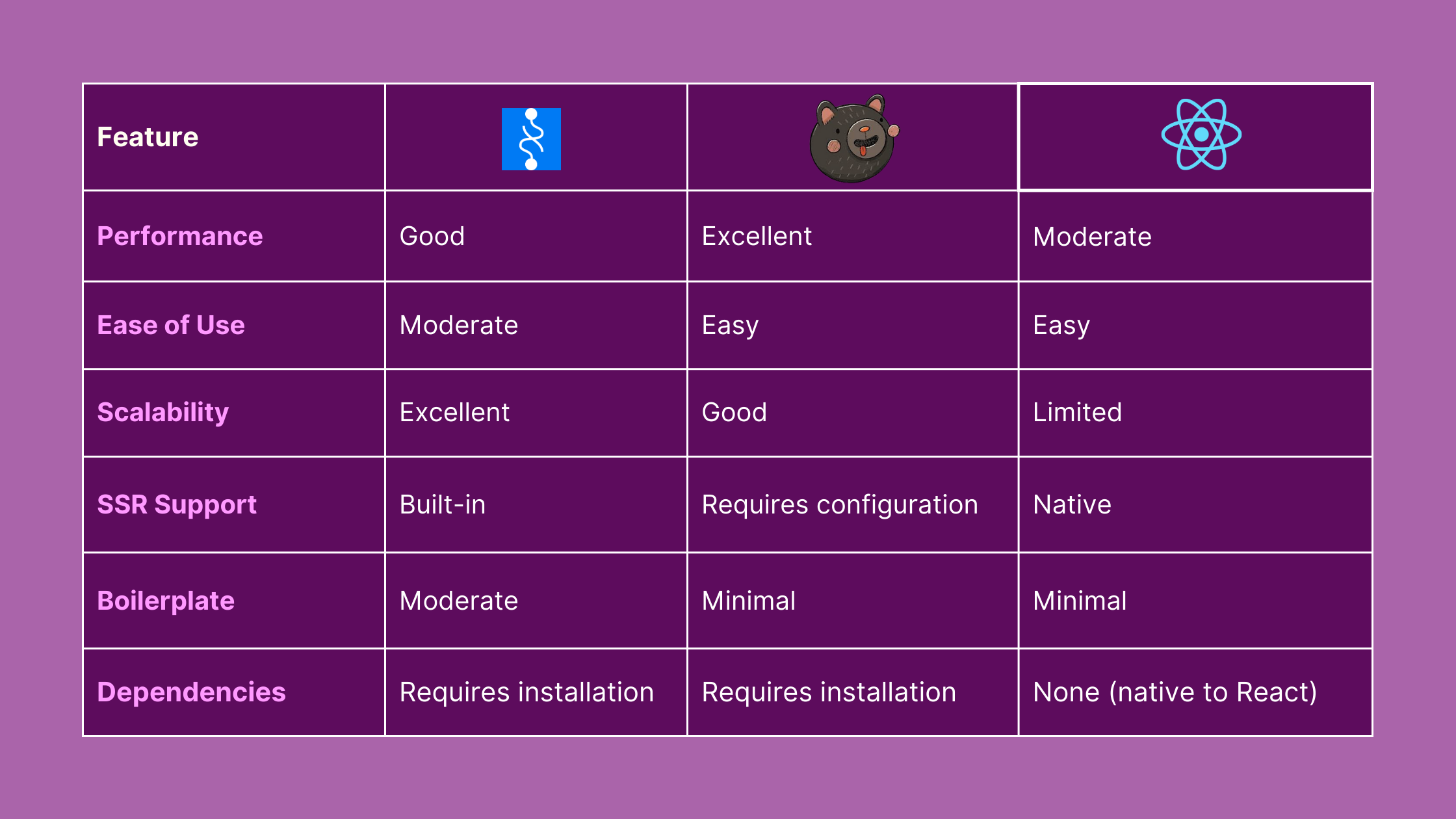
Which One Should You Choose?
The choice depends on your project's size, complexity, and specific requirements:
- Use Recoil if you need a scalable solution with fine-grained state management and SSR support for a complex application.
- Use Zustand if you prefer simplicity, performance, and a lightweight library for projects of any size.
- Use Context API if your application is small, straightforward, and you want to avoid external libraries.
Conclusion
Each state management tool has its strengths and weaknesses, and there’s no one-size-fits-all solution. For most modern Next.js projects, Zustand or Recoil will provide better performance and scalability than the Context API, but Context API remains a solid choice for simple applications.
If you are building something new and spend too much time implementing a Figma design, you should check Polipo.
Would you like to try it?
Start here.